vuejs input Textarea binding with examples
The textarea
is a multi-line input component in HTML commonly used for form input in web applications, allowing users to provide detailed input.
You can create a textarea in HTML as follows
<textarea placeholder="Please enter comments"></textarea>
This post guide you through implementing two-way data binding for a textarea in Vue.js using v-model
and input binding
, providing practical examples.
Simple TextArea Example in Vue.js
For any form control, values of a textarea
are mapped to the Vue component instance using the v-model
directive. Vue’s two-way binding with v-model
facilitates the seamless transfer of data between the form input control and the controller.
In the Vue component below:
- The
textarea
is declared in the template. v-model
is added for two-way binding between the template and the script.- The message property is declared and configured in the
v-model
attribute within the data function.
<template>
<div class="hello">
<textarea v-model="message" placeholder="Please add"></textarea>
</div>
<span>Comments:</span>
<p style="white-space: pre-line">{{ message }}</p>
<br />
</template>
<script>
export default {
name: "textarea",
props: {
message: String,
},
data: function () {
return {
message: "",
};
},
};
</script>
<style scoped>
h3 {
margin: 40px 0 0;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
v-model
provides two-way data binding. The same result can be achieved with :value
and @input
(i.e., Textarea Input Binding):
:value
provides read the value from input ie one-way binding @input
allows setting value to an input text area.
This way we can achieve two-way binding without v-model
<template>
<div class="hello">
<textarea
:value="message"
@input="message = $event.target.value"
rows="10"
cols="50"
></textarea>
</div>
</template>
<script>
export default {
name: "textarea",
};
</script>
Character Count with TextArea Component in Vue.js
Here’s an example of limiting the character count of a textarea to 100 characters, displaying an error message if the count exceeds 100. The example includes
- Adding a
keyup
event with an event handler function (characterCount) bound usingv-on
or:
. - Defining initial values for textarea properties, including character and pending character count.
v-model
holding the data property message for two-way binding.isError
as a boolean flag to check if the count is less than-1
and display the error color inred
.characterCount
method checks the character count entered in the text area. Here is a complete code:
<template>
<div class="hello">
<textarea
:keyup="characterCount"
v-model="message"
placeholder=""
></textarea>
</div>
<span></span>
<p v-bind:class="{ error: isError }">
Remaining Character Count :{{ pendingCount }}
</p>
<br />
</template>
<script>
export default {
name: "textarea",
data: function () {
return {
totalCount: 100,
pendingCount: 100,
message: "",
isError: false,
};
},
methods: {
characterCount: function () {
this.pendingCount = this.totalCount - this.message.length;
this.isError = this.pendingCount < 0;
},
},
};
</script>
<style scoped>
h3 {
margin: 40px 0 0;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
textarea {
height: 150px;
margin-bottom: 5px;
}
.error {
color: red;
}
</style>
Output:
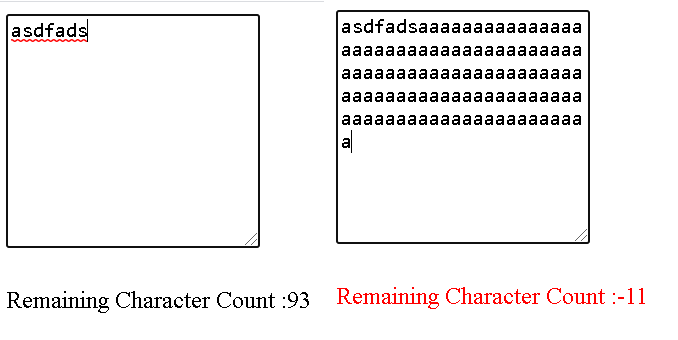