ES6 String Methods with examples in javascript| Learn Es6 tutorials
- Admin
- Jun 9, 2024
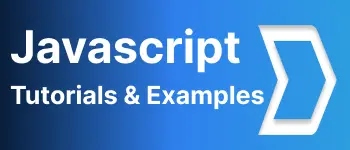
In this tutorial, we will explore EcmaScript 2015 String methods: startsWith(), endsWith(), includes(), and repeat(), with examples.
ES6 String Methods in JavaScript
ES6
introduced new methods for the String type object. In this blog post, I will cover these new string methods with examples. The newly introduced String methods can be generic and Unicode methods.
Generic Methods:
- startsWith()
- endsWith()
- includes()
- repeat()
JavaScript ES6 String.prototype.startsWith() Method Example
The startsWith()
method searches for a string match from a given start index.
Returns true
if the passed string matches the search string from the starting index position. If the index position is specified, it checks at the nth position.
Returns false
if the search string is not matched.
Syntax:
startsWith(searchString, index)
The two arguments are:
- String to search: substring to be searched.
- Index position: it is the position from which the search starts, default is zero.
Example:
const message = "This is a new string method";
console.log(message.startsWith("This is")); // true
console.log(message.startsWith("Thias is")); // false
console.log(message.startsWith("is", 4)); // false
console.log(message.startsWith("is", 5)); // true
JavaScript ES6 String.prototype.endsWith() Method Example
The endsWith()
method matches another string from the end index. It also takes two arguments.
This method returns true if the string end matches with another string.
Syntax:
endsWith(searchString, index)
It has two arguments:
- Search string: string to search that matches from the end.
- Index position: it is a position to match with the string, this is optional.
Example
const message = "This is a new string method";
console.log(message.endsWith("method")); // true
console.log(message.endsWith("methodsa is")); // false
console.log(message.endsWith("is", 4)); // true
console.log(message.endsWith("is", 5)); // false
JavaScript ES6 String.prototype.includes() Method Example
This returns true if the search substring contains a string.
includes(searchstring,index)
It has two arguments:
- Search string: string to be matched with the given string.
- Index position: it is the starting position to begin searching the string, this is optional.
const message = "this is testing include";
console.log(message.includes("testing")); // true
console.log(message.includes("adfadf")); // false
console.log(message.includes("is", 8)); // false
console.log(message.includes("is", 2)); // true
JavaScript ES6 String.prototype.repeat() Method Example
This method repeats the given string a given number of times. It concatenates strings.
str.repeat(count)
Parameter: the number of times to repeat the string.
Example:
const message = "cloud";
console.log(message.repeat(2)); // cloudcloud
console.log(message.repeat(3)); // cloudcloudcloud
JavaScript ES6 Unicode Methods
ES6 introduced methods for dealing with Unicode strings. Here are the methods:
- String.fromCodePoint: Returns Unicode.
- String.prototype.codePointAt.
- String.prototype.normalize.
Conclusion
Learn new string methods examples: startsWith(), endsWith(), includes(), and repeat().